You are thirty-six questions away from hiring your ideal Node.js developer
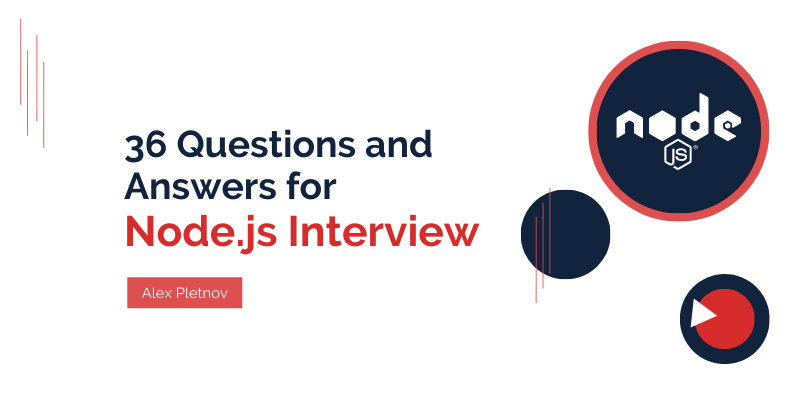
Node.js is a popular server-side platform using which one can build everything, starting from a simple command-line tool to a complex enterprise-level app. It’s a sound reason why there is a demand for an experienced Node.js Developer.
We’ve compiled a list of Node.js interview questions and answers to expect. Based on our experience, we came up with two good practices. Firstly, interview questions on Node.js aren’t the only ones you should ask. Secondly, to make a welcoming and successful hiring process, it’s reasonable to divide Node interview questions into 7 blocks:
- General programming
- JavaScript
- Database
- Node js questions
- Network data transfer
- Security
- Code testing
We’ll explain why each block is important for the developer to know. Under each question, you’ll find the approximate answers you should expect to receive.
Programming Basics
Why there’s a need to ask these Node.js interview questions? Node.js development is never exclusively about Node.js. Sooner or later, the developer will have to deal with other technologies, programming approaches, and challenges. The level of basic programming knowledge shows how prepared the candidate is to broaden their professional horizons. Let’s start.
1. What are the pros and cons of monolithic and microservice architecture?
What answer to expect:
Monolithic architecture is easier to work with as it’s linear and more fixed than microservices architecture. At the same time, monolithic components are tightly coupled, which complicates scalability, management, and continuous deployment.
Microservice architecture is more complex and more challenging to work with. The performance of such apps is also poorer because the communication between microservices requires more resources. However, this architecture is easy to scale and fast to develop.
2. What sorting algorithms are you familiar with, and how do they differ?
What answer to expect:
There are a lot of different algorithms, such as selection sort, bubble sort, quick sort, insertion sort, or merge sort. Each of these approaches has its principle, its algorithm, as well as its benefits and drawbacks. For instance, selection sort is looking for the minimum element from the unsorted part and placing it at the beginning of the array. Meanwhile, bubble sorting is looking at two neighbor elements, comparing them, and ordering the two of them.
3. How do you measure the complexity of a sorting algorithm? Have you ever had a chance to optimize algorithms?
What answer to expect:
The complexity of algorithms is measured by the amount of time they take to complete and the amount of memory they require. The complexity of sorting algorithms is marked as O, and there are different types of it:
- O(n) — linear complexity (for example, maximum element search algorithm),
- O(log n) — logarithmic complexity (for example, binary search),
- O (n^2) — square complexity (for example, insertion sort).
4. What is the difference between horizontal and vertical scaling?
What answer to expect:
Horizontal scaling stands for extending the pool of resources by adding more machines. Vertical scaling stands for extending the resources of existing machines by adding more power to them, for instance, advancing CPU or RAM.
5. What do you know about ACID, CAP, and SOLID patterns? Have you got a chance to use them on actual projects?
What answer to expect:
The ACID pattern stands for a set of properties of database transactions, which includes atomicity, consistency, isolation, durability. The CAP theorem, also called Brewer’s theorem, states that a distributed data store can simultaneously provide not more than two out of the three following principles: consistency, availability, and partition tolerance. SOLID is used in object-oriented programming, and it stands for single responsibility, open-closed, Liskov substitution, interface segregation, and dependency inversion.
The actual experience is also significant, so it’s good if the candidate has had a chance to apply these theorems in practice.
6. When is it better to use inheritance over composition?
What answer to expect:
Inheritance and composition are two programming principles developers use to model relations between classes and objects. Both concepts determine the app’s design and show how the app should function and develop with newly implemented features and changes. Inheritance and composition allow the reuse of code but in different ways. Composition determines a class as the sum of its parts, whereas inheritance separates one class from another. Through inheritance, classes and objects are closely linked. If to change the parent or superclass, you can break your code. Classes and objects are loosely coupled in composition, and it’s easier to change component parts without breaking the code. Because of flexibility, many developers consider composition a better technique.
JavaScript Basics
Why there’s a need to ask these Node.JS interview questions? Node.js is the technology that enabled the development of the back-end with JavaScript. You can’t work with a JavaScript runtime environment without knowing JavaScript itself. After all, everything always starts with the basics.
7. What is “this” keyword, and what does it refer to? What is the difference between “this” and context?
What answer to expect:
In JavaScript, the keyword “this” refers to the object it belongs to. It refers to a global object if located alone or in a function, to a local object if located in a method, or to an event if located in an event. Context also refers to objects, but it refers to the object located inside the executed function, while “this” refers to the object the function is executed in.
8. What’s a promise, and why was it introduced?
What answer to expect:
Before answering, it’s relevant to mention that handling asynchronous operations is complex. JavaScript offers different options to handle it, including callback, promises, rxjs, and async/await. Callback hell is one of the problems we sometimes face. The issue appears when we have many asynchronous operations, and we can’t chain them. A callback isn’t perfect. By handling multiple asynchronous functions, it can turn our code into something complex and not readable – and that’s an answer you may expect from most candidates.
More experienced developers will answer differently.
What answer to expect:
Promises solve two problems:
1. Inversion of control. We can’t control how many times a callback is called. There can be a callback for success or failure, or both at once. A promise can impose certain constraints on it.
2. While with callbacks, different libraries organized their work in their own way, promises standardized the interface. Over time, this paved the way for the async/await implementation.
A promise is a good alternative. It’s the final result of an asynchronous operation. The promise performs two functions: resolve and reject. By choosing resolve, we complete and send back the promise. By choosing reject, we can find or throw an error. Working with promise is not so complex. Promise helps us do dot-chaining. We can adjust any parameter to getUserData() we need. Using then(), we can accomplish any function. Cath() allows us to better handle errors and detect any rejects.
9. What is bind(), call(), and apply()? What is the difference between call() and apply()? Can you write your own version of the bind() function using apply()?
What answer to expect:
Call() is a method invoking a function and enabling the passing of arguments one by one. Apply() is a method invoking a function and enabling the passing of arguments as an array. Bind() is a method returning a function, which will be executed later with the correct context.
The technical example of the bind() function written using apply() is one of those Node js exercises depending on the candidate’s experience and understanding. The solution may differ, but it’s important the candidate can provide one.
10. What is the difference between regular functions and arrow functions?
What answer to expect:
Regular functions are quite ordinary, but arrow functions have been introduced recently by ES6 to enable writing concise JS functions. Regular functions are constructible as they can be created with a function declaration or expression. Also, they are callable because they can be called with a “new” keyword. Meanwhile, arrow functions are callable but not constructible. Also, unlike regular functions, arrow functions don’t have “this” keyword and don’t have the arguments keyword inside them. Arguments objects are also available only in regular functions.
Database Basics
Why there’s a need to ask these Node JS developer interview questions? Back-end development refers to the server-side of a software product — it regulates the communication between a database and a browser. In JavaScript, the questions on back-end development equal the questions on Node.js, so there is no need to create a separate block of questions on the back-end. However, there is a need to see if the developer knows how to work with databases.
11. What is the difference between relational and non-relational (SQL and NoSQL) databases? What needs does each type suit best?
What answer to expect:
Relational databases, such as PostgreSQL and MySQL, store data in tables. Non-relational databases, such as MongoDB, store data in JSON documents. Relation databases are best used when your app has to support dynamic queries. Meanwhile, non-relational databases are the best choice if you prioritize easy scalability.
12. What levels of database normalization are there? To which level, in your opinion, should you normalize your database so that it is acceptable?
What answer to expect:
There are three basic levels of database normalization: first, second, and third normal form, abbreviated 1NF, 2NF, and 3NF accordingly. In the database of 1NF level, data is stored in a relational table, each column contains atomic values, and no groups of columns are repeated. The 2NF database meets all the conditions of the first normal level, plus all the columns depend on the table’s primary key. The third normal level adds another condition — all the columns shouldn’t depend transitively on the primary key. After these, there goes BCNF — Boyce–Codd normal form, also known as 3.5 normal form. To be of a BCNF level, the table should be 3NF and have a super key for A in every dependency A → B. There’re also fourth and fifth normal levels, but they are more advanced. A 4NF table is the one following BCNF and has no multivalued dependencies. A 5NF table should be of 4NF and have no lossless decomposition into any number of smaller tables.
As for the question “To which level should you normalize your database so that it is acceptable?” there is no right or wrong response. Yet, the candidate’s answer can show you how confident they feel about databases and how meticulous they are.
13. What types of JOINs are there in SQL? How do they differ?
What answer to expect:
There are five types of JOINs in SQL: inner, left, right, full, and cross. To explain the difference, imagine two circles, A and B, overlapping. Each circle represents a table, and the overlap represents the set of records present in both A and B tables. Inner JOIN is the area of overlap, that is, all the present data in both tables. Full JOIN is the area of both circles combined, that is, all the data present in Table A and Table B combined. Left JOIN is the area of Circle A, that is, all the data present in table A even if it’s also present in Table B. Respectively, right JOIN is the area of Circle B, that is, all the data present in Table B. Meanwhile, a cross join produces a cartesian product between both tables. It returns all possible combinations of all rows.
14. How do indexes work, and what are those?
What answer to expect:
Indexes are the values used to sort database records. An index contains a field value and a pointer to a specific record. Then, the indexes are sorted. Indexing speeds up the search in the database because it tells the app which field to look up, and the SQL engine doesn’t have to look through each and every row.
15. What do you understand by ‘Atomicity’ and ‘Aggregation’?
What answer to expect:
If a transaction follows the principle of atomicity, either all or none actions of this transaction will be performed. In other words, if the transaction wasn’t completed successfully, the database will undo all completed actions by itself.
Aggregation stands for a principle of the relationship being expressed with the collection of entities and their relationships.
16. Do you know what ORM is and what it is used for?
What answer to expect:
ORM stands for object-relational mapping. This technique enables you to convert data between incompatible type systems with the help of object-oriented programming languages.
17. What types of relations are you familiar with?
What answer to expect:
There are three types of relationships:
- One-to-one,
- One-to-many,
- Many-to-many.
The one-to-one relation means that a single record from one table can be related to a single record from the other table.
The one-to-many relation means that a single record from one table can be related to zero, one, or many records from the other table.
The many-to-many relation means that any records from one table can be related to any records from the other table.
Node Basics
Why there’s a need to ask these interview questions for Node JS? This block is the simplest one: when you conduct a NodeJS interview, you ask the most common Node.js interview questions.
18. How does asynchronicity work in Node.js?
What answer to expect:
Asynchronicity means that the following operation doesn’t wait for the previous one to be completed. The asynchronicity in Node.js works due to its event-based, non-blocking I/O model.
19. What is an event loop, and how does it work in Node.js?
What answer to expect:
The event loop monitors the call stack and the callback queue. If the call stack is empty, it takes the event that is the first one in the queue and pushes it to the call stack, where it will be run. This event loop iteration is called a tick, and each event is simply a function callback. The event loop consists of six phases: the incoming connections go through poll, check, close callbacks, timers, I/O callbacks, and idle and prepare.
20. What are the types of streams in Node.js, and how do they differ?
What answer to expect:
There are 4 types of streams in Node. js:
- readable streams,
- writable streams,
- duplex streams,
- transform streams,
- pass-through streams.
Readable streams serve as an abstraction of a data source from where the data is taken. Writable streams serve as an abstraction of a data destination where the data is written. Duplex streams are both readable and writable. Transform streams are the same as duplex streams, but they can modify or transform the data while reading or writing it. Pass-through streams are a more trivial implementation of transform streams. It simply passes the input bytes across to the output. It’s usually used for examples and testing.
21. What is the difference between process.nextTick(), setImmediate(), and setTimeout(() => {}, 0)? In what order will they execute and why?
What answer to expect:
process.nextTick() defers the execution of a function until the next iteration of event loop begins.
setImmediate() executes the scenario after the current poll phase is completed.
setTimeout(() => {}, 0) plans the scenario launch after the minimum time interval in milliseconds passes.
They will execute in the following order:
- process.nextTick(),
- setImmediate(),
- setTimeout(() => {}, 0).
22. What is child_process, and what can it be used for?
What answer to expect:
child_process is a module enabling accessing the functionalities of the OS by running any system command inside a child process.
The answer on what child_process can be used for will differ from the candidate’s experience. The point of this question is to see if they have experience working with it.
23. How does Node.js overcome the problem of I/O operations blocking?
What answer to expect:
The event-based model of Node.js solves the problem of blocking input-output operations and uses event loops instead of threads.
Network Data Transfer Basics
Why there’s a need to ask these full-stack Node JS developer interview questions? API is something both front-end and back-end developers have to work with. They must know the basics of network data transfer to avoid critical security errors.
24. How does HTTPS work? What are the types of SSL certificates?
What answer to expect:
HTTPS is an extension of HTTP (the Hypertext Transfer Protocol). It adds SSL/TLS layer on top of HTTP. An SSL certificate is a small data file with a cryptographic key activating the padlock and the HTTPS protocol to establish a secure connection between a web server and a browser.
There are 3 types of SSL certificates by the level of validation they provide:
- Domain Validation,
- Organization Validation,
- Extended Validation.
25. What are the main principles of REST?
What answer to expect:
There are 5 principles of REST:
- Contract first approach / Uniform Resource identifiers,
- Layered architecture,
- Statelessness,
- Client-Server model,
- Caching.
26. Are you familiar with SOAP, and how does it differ from REST?
What answer to expect:
SOAP stands for Simple Object Access Protocol, and REST stands for Representational State Transfer. Respectively, SOAP is a protocol, and REST is an architectural pattern. While SOAP works only with XML, REST works with plain text, XML, HTML, and JSON. Thus, SOAP can’t use REST, but REST can use SOAP. Finally, SOAP needs more bandwidth for its usage than REST.
27. Have you ever used message brokers, such as RabbitMQ, and what are they for? What benefits do they offer?
What answer to expect:
Message protocols are intermediary software modules. The aim is to translate a message from the sender’s formal messaging protocol to the receiver’s formal messaging protocol and help apps, services, and systems communicate with each other. Message brokers are used for microservices. When one microservice falls, the message is queued until the microservice works again. This way, no messages will be missed, and the security and reliability of the system will increase.
28. What is long polling?
What answer to expect:
Long polling (HTTP long polling) is when the server holds a client’s connection open until new data is available. In the original client/server model, the web client is always the initiator of transactions — it’s the one to request data from the server. The server can’t independently send data to the client until it receives a request. The long polling technique was introduced to solve this problem.
Security Basics
Why there’s a need to ask these interview questions for Node.js developers? Security is the number one concern of modern-day Internet users. It’s not optional but obligatory to keep your app safe and invincible. This is why you have to ensure your developers know what security threats to expect and how to prevent them.
29. What is an SQL injection, and how to prevent it?
What answer to expect:
SQL injection is a malicious attack on a database conducted by placing malicious code in SQL statements via web input. To prevent SQL injections, developers have to stop writing dynamic queries. Also, they should avoid user-supplied input containing malicious SQL from influencing the query logic.
30. Have you heard about a timing attack? What is it, and how to prevent it?
What answer to expect:
A timing attack is a security threat, where an offender discovers security vulnerabilities of the system by analyzing how long the system responds to different inputs. To prevent timing attacks, the comparison of strings shouldn’t depend on their length. Also, the system should analyze and compare all the characters of both strings before returning true or false.
31. How to avoid broken access control?
What answer to expect:
A broken access control attack is a security threat when an attacker gets unauthorized access to some parts of the website.
To avoid broken access control, you should identify and prevent the following issues:
- Access control checks: users should pass certain security checks before getting access to some other parts of the website, and they shouldn’t be able to bypass this check by simply skipping the page.
- IDs: web apps should not over-rely on id because guessing the id may grant an attacker unauthorized access.
- File permissions: files that aren’t to be presented to web users shouldn’t be marked as readable, let alone executable.
- Client-side caching: attackers can access web pages cached by browsers to gain unauthorized access.
32. What is server XSS?
What answer to expect:
Server XSS (cross-site scripting) is a security threat when unreliable user input is included in an HTML response generated by the server. This input can be sent from a request or a stored location. The server-side code is vulnerable when a server XSS occurs, and the browser renders this response. To prevent server XSS, you should encode context-sensitive server-side output.
33. What is a same-origin policy?
What answer to expect:
The same-origin policy is a critical security mechanism restricting the interaction between a document from one origin and a document from another origin. This way, you can isolate potentially dangerous documents and prevent security attacks.
Code Testing Basics
Why there’s a need to ask these Node.js interview questions for experienced developers? Before submitting code to the Quality Assurance team, the developer should run automated code tests — it greatly optimizes the software development process. It would be best to ensure the candidate knows how to do it.
34. What libraries do you use for unit, functional, and integration testing in Node.js? What do you write tests for?
What answer to expect:
The following libraries can be used: Mocha/Chai, Jest/Jasmine.
“What do you write tests for?” is a rather philosophical question with no correct answer to it, so it serves to show you the way the candidate thinks.
35. What are mocks and stubs? Why should we use realistic data for that?
What answer to expect:
Mocks and stubs are objects used in testing looking and behaving like actual production test objects, but they’re more simplified. Stubs are objects holding predefined data and using it to answer calls during tests. Mocks are objects registering calls they receive to verify that all expected actions are performed.
Yet, we should use accurate data to emulate the working environment and catch actual cases.
36. Is it a good idea to run test coverage for each pull request in your CI?
What answer to expect:
This question is also one of those philosophical Node js interview questions for experienced developers. The most obvious answer would be that you shouldn’t run test coverage for each pull request during a heated development process because it’ll take too much time. However, running full test coverage is a must when you’re releasing essential features.
In our blog, we have also discussed 36 React interview questions to ask an experienced developer you may be interested in.
To Wrap Up
This article is a win-win solution:
- If you are a developer, you are 36 answers away from getting a job as a Node.js developer.
- If you are the one to hire, you are 36 questions away from finding your perfect candidate.
But if you still feel like you need help or would like to trust us with the choice of Node.js developers, feel free to contact us.
Our Keenethics team will be ready to help you! Learn more about Node.js development services that we offer or contact us!